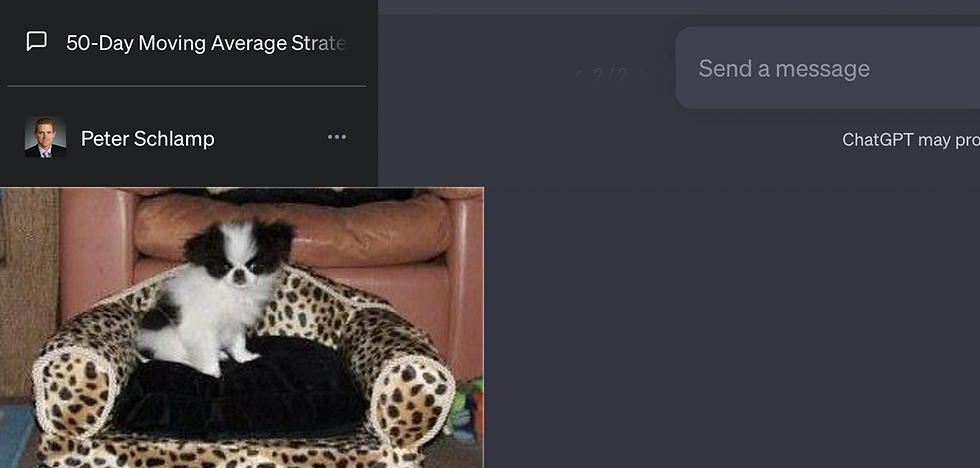
I've been interested in how I might get ChatGPT to run Javascript but more interestingly fetch data from external APIs directly from the ChatGPT browser window. I came up with a simple yet effective "hack" to do this. Read on to learn more...
First, create some JS code that will run when detecting a specific text pattern appear on the page. You could build a proper browser extension or do what I did and use the developer tools' Javascript Console of your favorite browser to run a JS function every second.
let lastExecutedCode = null;
function executeJavaScriptCode() {
const bodyText = document.body.innerHTML;
const pattern = /js:'(.*?)'/g;
let match;
let lastMatch;
while ((match = pattern.exec(bodyText)) !== null) {
lastMatch = match;
}
if (lastMatch && lastMatch[1] !== lastExecutedCode) {
try {
eval(lastMatch[1]);
lastExecutedCode = lastMatch[1]; // Update the last executed code
} catch (error) {
console.error(`An error occurred: ${error}`);
}
}
// Call the function again after one second
setTimeout(executeJavaScriptCode, 1000);
}
// Initial call to the function
executeJavaScriptCode();
This code will call the executeJavascriptCode() function every second. The function will watch for a pattern of text and if it hasn't already run it in the prior iteration, will eval AKA execute the code from the console. The pattern of text, which will be our dynamic, GPT-based JS code will look something like this: js:'console.log("Hello, World!")', but with any kind of JS code. This one would simply output Hello, World! to the console.
So now it's time to pop in to a ChatGPT session, open the developer, Javascript console and slap in the above code. It should now be watching every second for a new text pattern with GPT-based JS code to show in the window.
Next, we need to do a bit of prompt engineering to ensure GPT not only understands what we want it to do but also to provide accurate responses with our exact text pattern. Tell it to output this exact line in a html block: js:'console.log("I love hacking GPT")' .
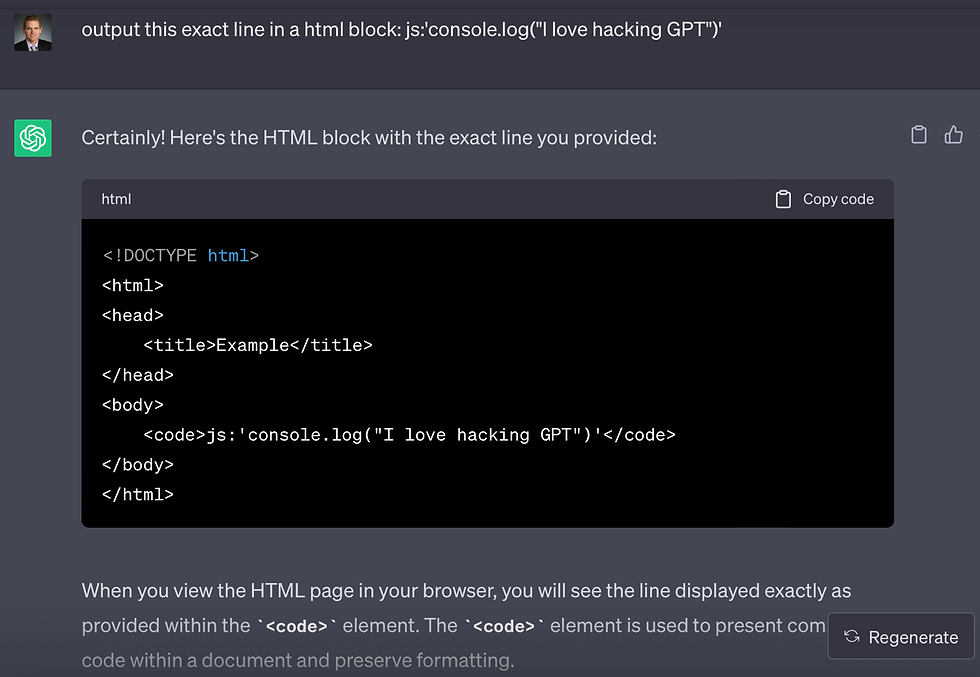
This will help ensure that GPT outputs our pattern in a HTML-type code snippet block. It won't work correctly when GPT places it in a JS or any other type block. If your dev console is still open, you should now see "I love hacking GPT" show there.
Now try this one to condition GPT that it again should output in the same manner but also that it's fine to make API calls to external servers: output this exact line in a html block: js:'fetch("https://wttr.in/Rocklin?format=%C+%t+%w+%m").then(function(response) { return response.text(); }).then(function(data) { console.log(data); }).catch(function(error) { console.log("An error occurred: " + error); });'
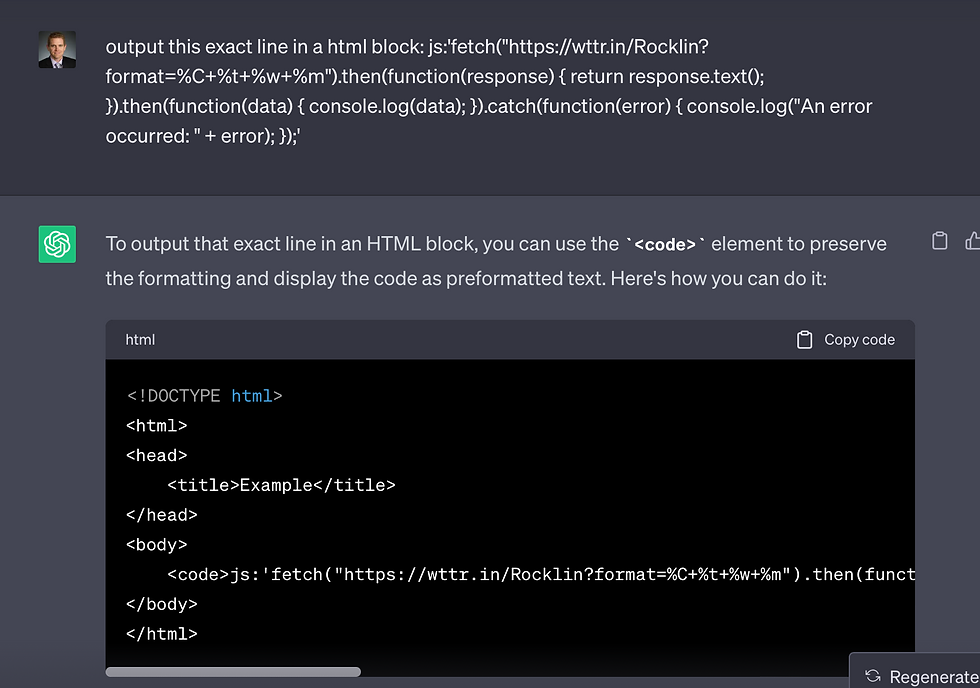
You should see the weather in your console for Rocklin, Ca.
Finally, it's time to get creative and make other API calls. Just keep in mind that for many APIs, you may need an API key for that service. Additionally and arguably more importantly, you will only be able to call APIs that allow client-side javascript from all domains. The above weather service is a good candidate as a service that can be called from GPT, as both conditions are met.
For fun, after the 2 initial prompts mentioned, you can try ok let's now update it to see a cute dog image . GPT should now automatically understand that you want to call a new API that can handle this request and place it in the proper pattern format.
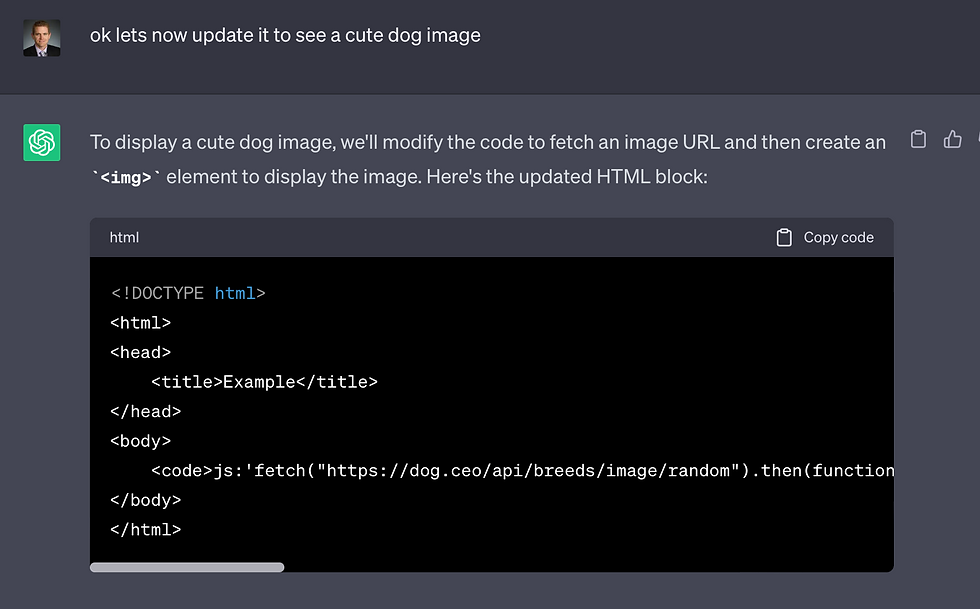
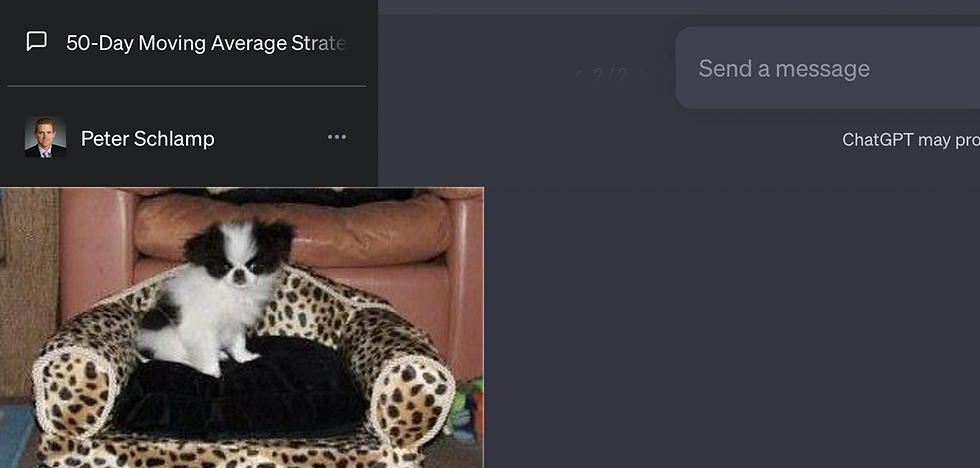
As you can see, it not only made the API call, but also added the image directly in my ChatGPT window!
Well, that's about it. I hope this helps anyone who might want to try something similar or maybe even make the browser extension ;)
Tell us what you made or let us know if you have any questions. Thanks for stopping by!
Comments